Signet's Execution Engine
Signet uses a straightforward order system for cross-chain transactions. We remove unnecessary verification layers to deliver faster settlements with lower costs. Our architecture focuses on reliable asset transfers between networks without technical complexity.
The order system uses signed orders to describe a user’s intent and bundles to coordinate execution of these intents across chains.
Conditional transactions via signed orders
Signed orders use the Permit2 standard, allowing users to authorize token transfers with a single signature. This intent mechanism powers Signet’s swaps, allowing users to express intents like:
“I want to swap 1 ETH on Signet for 1800 USDC on Ethereum”
Nothing happens if the exact conditions you specify are not met.
1// Signed orders
2pub struct SignedOrder {
3 /// The permit batch.
4 #[serde(flatten)]
5 pub permit: Permit2Batch,
6 /// The desired outputs.
7 pub outputs: Vec<Output>,
8}
9
10// Permit2 batches
11pub struct Permit2Batch {
12 pub permit: <PermitBatchTransferFrom as SolType>::RustType,
13 pub owner: Address,
14 pub signature: Bytes,
15}
Permit2 batches contain individually signed transactions, allowing multiple inputs (what the user provides) to map to desired outputs (what the user receives). These Permit2 transactions then go to an orders cache for matching.
Cross-chain transfers
Signet’s approach to cross-chain transfers separates mechanisms based on direction. This separation creates predictable pathways that optimize for each direction’s unique requirements.
Entering Signet: Passage
For asset transfers from Ethereum to Signet, users will interact with the Passage contract, which follows a simple event-based approach:
- User deposits assets to the Passage contract on Ethereum
- The contract emits an Enter event
- Signet observes this event and mints corresponding tokens
Exiting Signet: Signed Orders
For asset transfers from Signet to Ethereum, users will use signed orders (swaps).
- Signed orders express user intent to move assets from Signet to Ethereum
- Market participants fulfill these orders with corresponding assets
- Execution happens atomically in the same block on both chains
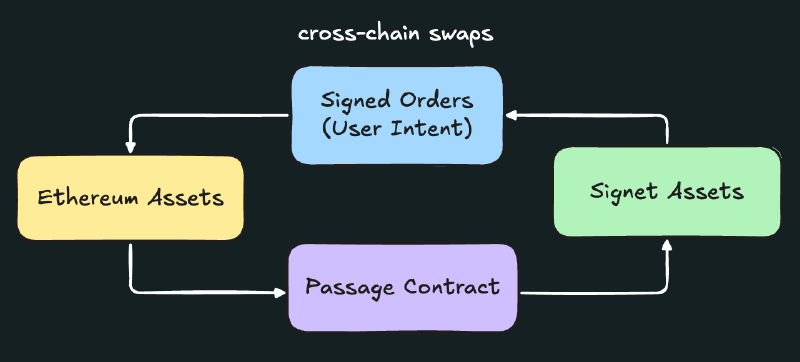
Market Participants
Our market-based approach eliminates the need for verification periods or complex bridging. Users move assets instantly between chains.
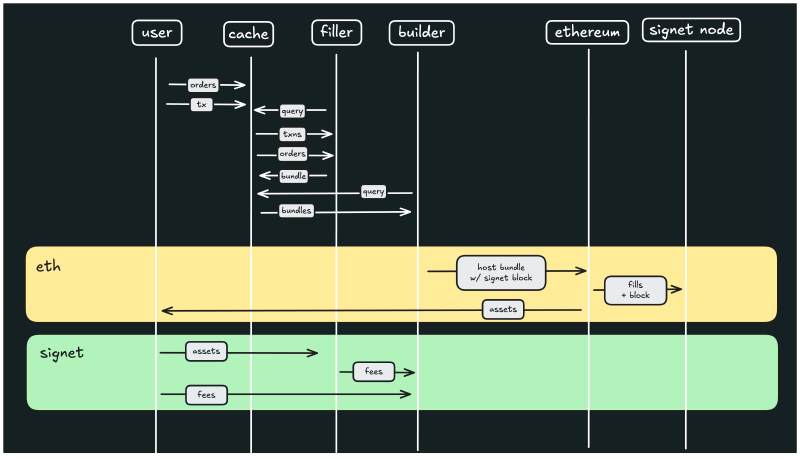
Searchers
Searchers connect orders with liquidity and are essential for creating efficient markets. They:
- Scan orders cache
- Build matching permits into bundles
- Submit these bundles to block builders
- Drive efficiency by connecting orders with liquidity
Fillers
Fillers provide liquidity to the system. They:
- Fulfill open orders with their own assets
- Enable cross-chain transfers without traditional bridges
- Make instant withdrawals possible
Block Builders
Block builders assemble transactions into Signet blocks. They process two primitive types – bundles and signed orders.
- Bundles are standard collections of transactions to be included in blocks. 4844 transactions are excluded from the rollup by design.
- Signed Orders are permit2 transactions expressing user intents. These connect cross-chain activity via
hostFills
.
Bundles coordinate cross-chain actions
We extend Flashbots bundles with a single field: hostFills
. This connects Signet transactions with Ethereum actions:
1{
2 "txs": ["0xabc...", "0xdef..."],
3 "blockNumber": "0x123",
4 "hostFills": {
5 "outputs": [...],
6 "permit": {
7 }
8 }
9}
This extension enables atomic cross-chain execution — the Signet transactions and the necessary host chain actions either all succeed together or the entire bundle fails.
A Signet bundle includes:
- An array of transactions (
txs
) - Block targeting information (
blockNumber
,minTimestamp
,maxTimestamp
) - Optional
revertingTxHashes
identifying Signet transactions allowed to revert without failing the whole bundle. - Optional
replacementUuid
for identifying updated bundles - The
hostFills
field containing required host chain actions (if any).
Bundles come in two forms:
- Bundles with host fills contain signed orderes requiring validation. The bundle builder tracks and validates these orders. Host fills are processed alongside bundle transactions.
- Bundles without host fills handle standard in-network activity. These contain regular Signet transactions and are processed like standard transaction lists.
When building systems that handle real value, reliability isn’t optional. That’s why we’ve adopted flashbots-style bundle standards:
- Transactions execute exactly as specified
- All operations succeed or none happen
- Transactions only revert when explicitly marked as revertible
Build with us
We’re building the simplest and most pragmatic rollup. If you’re interested or have feedback, please reach out: